iPhone Apps
A collection of simple iPhone Apps built as part of a course to learn more about Swift and iOS app design

Xylophone App
In this app project, I got the chance to learn to write Swift code to enable buttons or objects to change (increasing transparency when pressed) and to call an action to play a sound. The result is a little xylophone.
Sample code:
@IBAction func keyPressed(_ sender: UIButton) { playSound(soundName: sender.currentTitle!) sender.alpha = 0.5 DispatchQueue.main.asyncAfter(deadline: .now() + 0.2) sender.alpha = 1.0 }
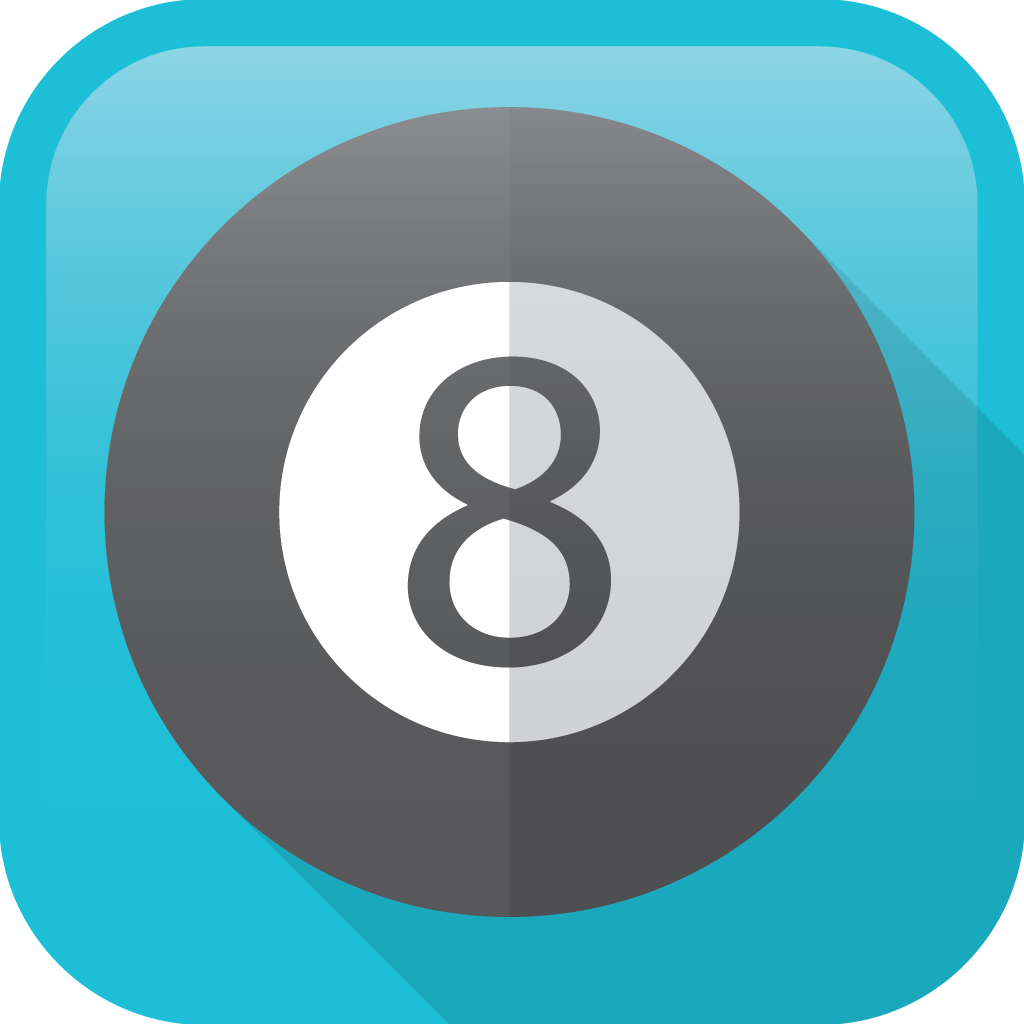
Not-So-Magic 8-Ball App
To create our own magic 8-ball, we used arrays and a random element operator to display an answer once you press the ask button.
Sample code:
@IBOutlet var viewBack: UIView! @IBOutlet weak var buttomView: UIButton! @IBAction func askButtonPressed(_ sender: UIButton) { imageView.image = ballArray.randomElement()

Dice rolling app
The dice rolling app is similar to the previous app, it’s simply a button linked to action to select a random image from an array for the two dice image.
A sample of the code below shows two different ways to call a random element from the array, one using a range and the other using the built in function .randomElement()
Sample code:
@IBAction func rollButtonPressed(_ sender: UIButton) { let diceArray = [ imageLiteral(resourceName: "DiceOne"), imageLiteral(resourceName: "DiceTwo"), imageLiteral(resourceName: "DiceThree"), imageLiteral(resourceName: "DiceFour"), imageLiteral(resourceName: "DiceFive"), imageLiteral(resourceName: "DiceSix")] diceImageView1.image = diceArray[Int.random(in: 0...5)] diceImageView2.image = diceArray.randomElement()